Test driven development is something which has helped me write better, cleaner and more modular code. In search of more coverage, I found PCOV which is a great tool to find a test coverage report. It is a great way to get a sense of how much code coverage we have and which situations are not getting covered. I will also talk about the internals of how PCOV work and detect the coverage.
Intro
I've always been a fan of test-driven development (TDD) because it makes coding more disciplined and ensures every function is covered from the get-go. TDD has helped me craft cleaner, more modular, and independent code by focusing on clear, testable outcomes. However, as my projects grew, I wanted even more coverage to ensure every line of code was tested. That’s when I discovered PCOV, a sleek and efficient code coverage tool for PHP. Integrating PCOV into my workflow gave me deeper insights into my test coverage, boosting my confidence in the reliability and robustness of my releases.
What is PCOV?
So, the first question that might come to mind is: What is PCOV?
PCOV is a code coverage driver for PHP, designed to help you see which parts of your code are being tested. Think of it as a tool that highlights the sections of your codebase that your tests touch and, more importantly, those they don't. It runs as a PHP extension and integrates seamlessly with PHPUnit. When you run your tests, PCOV generates a detailed code coverage report, showing you exactly which parts of your codebase need more testing love. This insight is invaluable for improving your test coverage and ensuring your code is as reliable as possible.
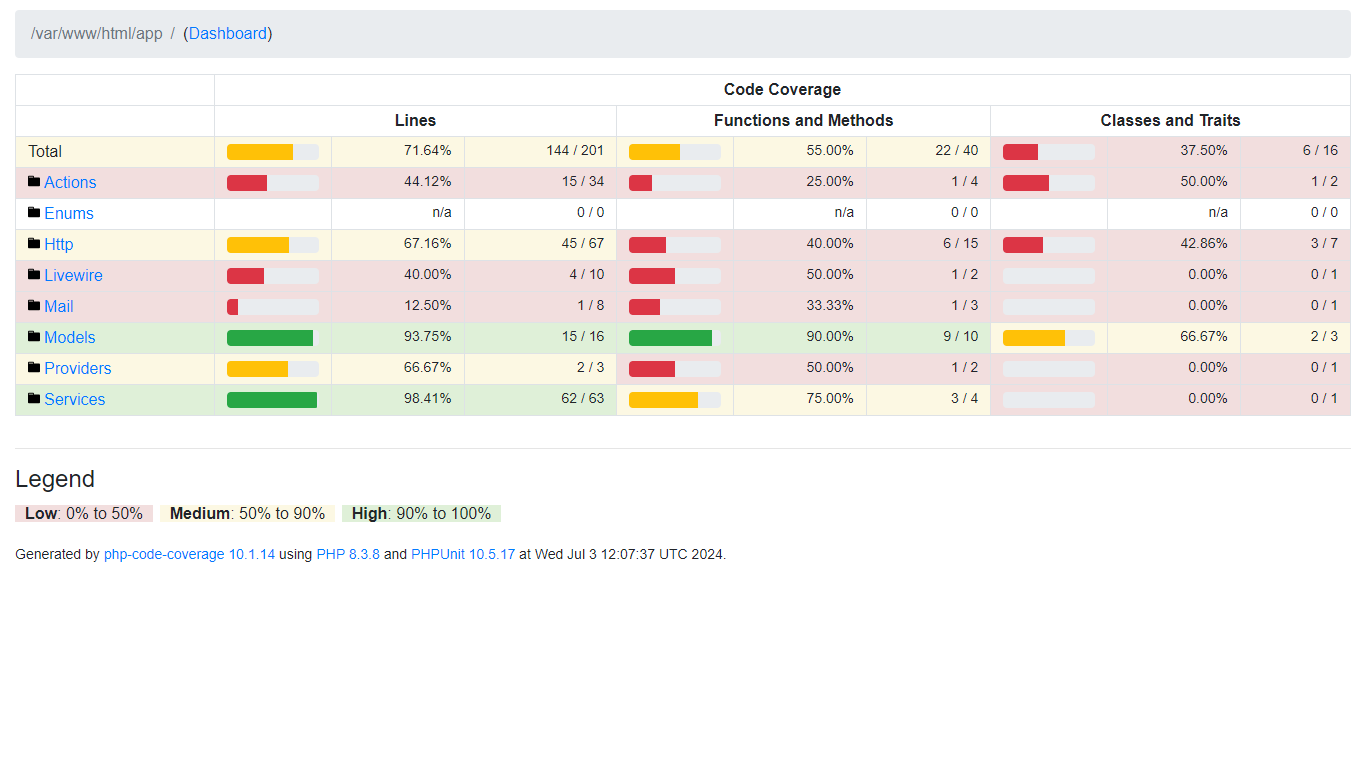
And how code coverage works?
Code coverage measures how much of your source code is executed when running a test suite. The main types of code coverage include:
- Line Coverage: Tracks which lines of code were executed.
- Function Coverage: Identifies which functions or methods were called.
- Branch Coverage: Determines which branches of control structures (like if statements) were taken.
To dive a bit deeper, let's understand how PCOV operates internally.
When PCOV is enabled, it instruments the code by adding monitoring hooks into the PHP engine's execution flow. These hooks track when a line of code, function, or branch is executed. As tests run, these hooks collect coverage data in memory. Once the test suite has fully executed, a comprehensive report is generated, showing detailed coverage information.
How I implemented it inside my Docker setup?
To use the power of this amazing tool inside my own Docker setup for a Laravel application which you can find here I installed the PHP extension inside my Docker container. I am using the Server Side Up docker container. It is very easy to install a PHP extension to this Docker container. Here is part of the snippet for you to understand
This is how I get the extension. And then I just had to make some configuration to my phpunit.xml
. Here is the configuration
With this, whenevery I run php artisan test
, my tests get executed and the report is regenerated.
What are the advantages of this report?
This report helps me get a visual representation of my code coverage and where I am lacking in terms of 100% code coverage. It tells me which classes are lacking the coverage and in those classes which methods are not getting covered. It even tells me which branch of the code is not getting covered. This allows me to cover all the business logic that I have ensuring a full coverage of my code using tests.
Why this is not the perfect report?
While achieving 100% test coverage according to PCOV reports is commendable, it's crucial to recognize that it's just a metric. Just as writing tests doesn't guarantee bug-free code, 100% coverage doesn't necessarily mean every aspect of your business logic is thoroughly tested. Consider a method that returns different arrays based on a conditional check, like this example:
PCOV might show full coverage if your tests cover both 18+
and <18
scenarios, but it won't capture nuances such as messages displayed to users. This highlights the importance of manual verification and thorough testing beyond basic coverage metrics. Remember, effective testing involves not just quantity but also quality, ensuring all critical paths and edge cases are thoroughly validated.
Conclusion
Even with this limitation, I can confidently say that PCOV is a fantastic tool for generating coverage reports. While 100% coverage doesn't mean every single aspect is tested, it serves as a valuable guide.
What do you think? If you have any questions, feel free to ask in the comments below or reach out to me on my social media handles.

Transforming ideas into impactful solutions, one project at a time. For me, software engineering isn't just about writing code; it's about building tools that make lives better.